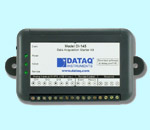 UltimaSerial
Windaq Add-ons
UltimaWaterfall
XChart
FFT1024
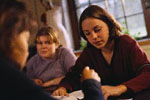
Lessons
|
|
If you just need to find a way to retrieve data files from a DATAQ
DI-71x SD with corrupted file system, check out Windaq
SD Rescue.
If you need to write a program to read the raw data from a damaged
SD's sector directly to see how bad the problem, here is a VB sample. It contains one text input for entering
the drive letter of the SD, and a command button to open and read the boot
sector of the SD.
Private Declare Function SetFilePointer Lib "kernel32.dll" (ByVal hFile As Long, ByVal lDistanceToMove As Long, _
lpDistanceToMoveHigh As Long, ByVal dwMoveMethod As Long) As Long
Private Declare Function CreateFileNS Lib "kernel32.dll" Alias "CreateFileA" _
(ByVal lpFileName As String, ByVal dwDesiredAccess As Long, _
ByVal dwShareMode As Long, ByVal lpSecurityAttributes As Long, _
ByVal dwCreationDisposition As Long, ByVal dwFlagsAndAttributes As Long, _
ByVal hTemplateFile As Long) As Long
Private Declare Function ReadFileNO Lib "kernel32.dll" Alias "ReadFile" _
(ByVal hFile As Long, lpBuffer As Any, ByVal nNumberOfBytesToRead As Long, _
lpNumberOfBytesRead As Long, ByVal lpOverlapped As Long) As Long
Private Declare Function CloseHandleNS Lib "kernel32" Alias "CloseHandle" (ByVal hObject As Long) As Long
Const GENERIC_READ = &H80000000
Const FILE_SHARE_READ = &H1
Const OPEN_EXISTING = 3
Const FILE_ATTRIBUTE_NORMAL = &H80
Dim hFile As Long
Private Sub Command1_Click()
Dim lLong As Long
Dim hLong As Long
Dim retval As Long
Dim longbuffer As Long
Dim numread As Long
Dim stringbuffer As String
lLong = 0
hLong = 0
HDRIVE$ = Left$(UCase$(Text1.Text), 1)
If HDRIVE$ < "D" Then
MsgBox "Sorry, but I think this drive letter is too low to be a SD drive"
Exit Sub
End If
hFile = CreateFileNS("\\.\" & HDRIVE$ & ":", GENERIC_READ, FILE_SHARE_READ, 0, OPEN_EXISTING, 0, 0)
l = SetFilePointer(hFile, lLong, hLong, 0)
stringbuffer = Space(512) ' make enough room in the buffer, SD's minimum
read size is 512 bytes
retval = ReadFileNO(hFile, ByVal stringbuffer, 512, numread, 0)
If numread = 0 Then ' EOF reached
Debug.Print "End of file encountered -- could not read any data."
Else
Debug.Print "String read from file: "
+ stringbuffer
End If
CloseHandleNS (hFile)
End Sub
Note: Be careful when using hex numbers in SetFilePointer, VB will
treat numbers between &H8000 and &HFFFF as a negative number, but
&H1FFFF backs to a positive number, and may screw up the offset. To
avoid confusion, use decimal numbers
Last update: 02/29/12
Copyright: 2000-2005
www.UltimaSerial.com
|